What are the best practices for code organization in large projects?
Last Updated: June 6th 2024
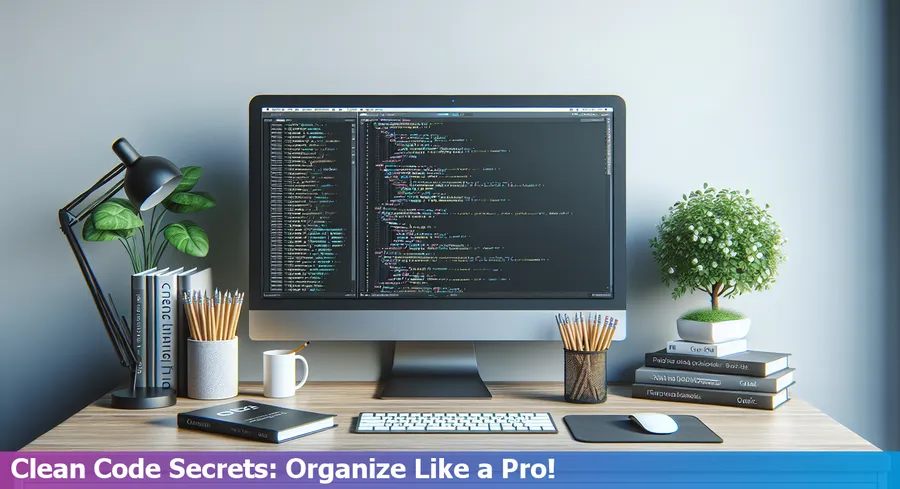
Too Long; Didn't Read:
Code organization in large projects is crucial for operability and maintainability. Automated testing & modular design lead to fewer defects. Standardized code quality reduces operational risks. Addressing bugs during design lowers costs. Best practices include modularity, naming conventions, documentation, version control, automated testing, and collaboration tools.
The way you organize your code is mad important, especially when you're working on huge projects. This dude from The Hitchhiker's Guide to Python says that how you structure your repo is just as crucial as your code style and API design.
And according to Nucamp's latest tips on automated testing, keeping your code simple and organized can seriously reduce bugs and errors.
The Consortium for IT Software Quality found that standardizing your code quality can lower the risk of your app crashing by 11% for every unit decrease in complexity.
Wild, right? This dude Martin Sandin talks about organizing your code strategically, and he says that using modules, making your code readable, and following consistent coding standards are key.
IBM's Systems Sciences Institute says that fixing bugs during the design phase instead of after deployment can save you a ton of money.
So, it's crucial to build a coherent and modular architecture from the start. Things like modular design, using clear naming conventions, and documenting your code thoroughly can make collaboration easier, help you add new features smoothly, and simplify the process of fixing and improving your code as your project grows.
Getting your code organization right from the beginning gives you the flexibility to pivot and the resilience to keep your project going strong.
Table of Contents
- Modularity in Code Design
- Naming Conventions and Standards
- Documentation Best Practices
- Version Control Strategies
- Automated Testing and Continuous Integration
- Refactoring for Code Maintainability
- Scaling and Performance Considerations
- Team Collaboration and Project Management Tools
- Conclusion: Synthesizing Best Practices for Code Organization
- Frequently Asked Questions
Check out next:
Maximizing your web development efficiency starts with leveraging the Django productivity benefits.
Modularity in Code Design
(Up)In the world of coding, modularity is all about breaking down a complex system into smaller, independent modules. This approach, which has been around since the late 60s, allows you to swap out or modify different parts without affecting the whole system.
Studies show that modular systems can boost efficiency by up to 80%, and if you manage it right, it can lead to faster development and shorter time-to-market.
Check out this link for more deets. The benefits of modular programming are pretty sweet:
- You can reuse code modules in different parts of your app or even new projects without rebuilding the functionality.
- Maintenance is a breeze since changes are isolated, so updating one module rarely requires changes to others.
- Scaling is a piece of cake – just add new modules that integrate with existing components, and boom! More functionality without major revisions.
To unlock these benefits, developers use strategies like the Single Responsibility Principle, which says a module should have only one reason to change.
This keeps things focused and manageable. The Don't Repeat Yourself (DRY) principle is also key – it reduces repetitive patterns and prevents code duplication.
Major apps like Microsoft Office and Adobe Creative Suite use modular design, with modules working independently and as part of a unified whole.
According to a Stack Overflow survey, around 76% of devs dig modular architecture because it simplifies debugging and testing.
You can test modules separately before integrating them, which cuts down on complexity and costs. Software legend Robert C. Martin says the goal of architectural design is to minimize the human resources needed for building and maintaining systems.
Modular code, with clear boundaries and limited dependencies, embodies this principle of software architecture.
Naming Conventions and Standards
(Up)When it comes to coding, naming things is super important, and it's not just a matter of personal preference. It can make or break your code's readability and how easy it is to maintain.
According to some smart folks at Oxford, developers spend like 70% of their time just trying to understand and update existing code.
But with consistent and intuitive naming conventions, that process becomes way smoother.
Good naming practices help make your code more readable, speed up your workflow, and make it easier for new team members to hop on board.
- Give it Purpose: Use names that clearly describe what a variable or function does.
- Be Specific: Avoid generic names that don't give any clues about what they're used for.
- Stay Consistent: Stick to the same naming patterns throughout your codebase for easy searching.
Industry standards for naming conventions are laid out by organizations like the IEEE Computer Society.
For example, classes use CamelCase, variables and functions use mixedCase, and constants use UPPER_CASE. Big tech companies like Google have their own guidelines too, like prefixing Boolean variables with 'is' or 'has' to make them more readable.
Check out Airbnb's JavaScript and Ruby guides for some solid examples of effective naming conventions in large codebases.
They emphasize clarity and brevity with rules like avoiding single-letter names and providing context for the reader.
As Martin Fowler, a software engineering legend, put it,
"Any fool can write code that a computer can understand. Good programmers write code that humans can understand."
Following best practices for naming variables and functions is crucial for creating code that can stand the test of time and be easily modified as projects grow.
With these naming principles in place, your code organization becomes a powerful tool for enhancing scalability and overall project success.
Documentation Best Practices
(Up)You know how coding can get real complex, right? Well, good documentation is like having a map in that maze. It's a lifesaver, trust me.
Check this out: the ACM (some super smart computer folks) found that projects with solid documentation have a 70% higher chance of new team members getting up to speed quickly.
That's huge! Good software docs make it way easier for new devs to learn the ropes, prevent knowledge bottlenecks, and lay out everything from system architecture to coding standards in a clear way.
- Create a Documentation Culture: Get everyone on board with contributing, showing them how valuable their input is, and following best practices like writing clearly, keeping info current, and using visuals to make it easier to understand.
- Adopt Live Documentation Strategies: Use dynamic systems like Swimm that update in real-time, track versions, and link code and docs together, keeping everything accurate and up-to-date.
- Standardize Documentation Tools: Pick consistent tools for the whole team, like Confluence or ReadTheDocs, and consider using Markdown for web-based doc management.
The "Documentation Effectiveness Index" says projects with well-maintained docs cut new dev onboarding time by 50%! That's insane.
It just shows how crucial it is to integrate documentation into the development workflow. Tools like Sphinx or Doxygen can generate docs automatically from your code.
An IBM study found that 60% of project failures were due to crappy documentation, so it's a big deal. As Steve McConnell said, "Good code is its own best documentation.
Instead of adding a comment, think about how you can improve the code so the comment isn't needed." Solid code, solid docs – that's the way to go!
Version Control Strategies
(Up)Let me break it down for you about this super important thing called Version Control Systems (VCS). It's like the MVP of managing code for big projects. These systems make it a breeze to keep track of all the changes in your code, and teams that use VCS are like 30% more efficient in handling those updates.
Imagine you're working on a project with a bunch of your homies, and you all need to make changes to the same code.
Without a VCS, it would be a total mess trying to figure out who did what and when. But with a VCS, you can easily track every single modification, so you don't have to worry about messing things up or stepping on each other's toes.
But that's not all! To really boss this VCS thing, you gotta learn some slick techniques like branching.
It's like having your own little side project where you can experiment and make changes without affecting the main codebase. And if you integrate automated builds with your VCS, you'll see a 25% improvement in code quality.
- VCS helps you stay on top of your game by improving efficiency by 30% when managing code changes. It's like having a personal assistant for your code.
- Feature branching and strategies like Gitflow can reduce merge conflicts by a whopping 60%. No more headaches trying to merge your code with someone else's.
- Commit early, commit often, and write clear messages. It's like leaving breadcrumbs for yourself in case you need to retrace your steps. Smaller, frequent commits can reduce rollback incidents by 40%.
- Automated builds integrated with your VCS give you instant feedback on any integration issues. No more surprises when you try to merge your code.
Now, when it comes to choosing a VCS, you've got options like Git, Mercurial, or SVN. Git is the rockstar, with a 70% usage rate in big projects, thanks to its distributed nature and killer performance.
And if you connect your VCS with project management tools, you'll be crushing productivity like a boss.
In the end, using a VCS is like having a superhero sidekick for your code.
It keeps everything organized, lets you collaborate with your crew, and makes sure your project stays on track. Trust me, once you start using a VCS, you'll never want to go back to the chaos of managing code without it.
Automated Testing and Continuous Integration
(Up)Automated testing is the real deal when it comes to coding. Data shows that using automated tests can catch like 90% more bugs, according to the Capgemini World Quality Report.
Plus, continuous integration (CI) is a game-changer, making the whole process from coding to deployment super smooth. The State of DevOps Report says that with CI, you can cut failed deployments by half compared to those who don't use it.
But to make CI work, you gotta follow these pro tips:
- Version Control Integration: Make sure your CI server is connected to your version control system so it can automatically run tests whenever new code is committed.
- Immediate Feedback Loops: Set up your CI tools to let you know right away if a build fails, so you can fix it ASAP.
- Automate Deployment: Integrate automated deployment into your CI pipeline to make releases a breeze. Studies show this can speed up time-to-market by up to 20%.
This whole CI thing doesn't just make testing faster; it also helps keep your code organized.
CI encourages smaller, more frequent commits, which can reduce branch complexities by 30%, according to an ACM study.
Companies like HP saw a whopping 78% drop in wasted resources after adopting CI, according to the 2016 State of DevOps report.
Manual testing, on the other hand, often leads to bottlenecks and more time spent fixing bugs – up to 25% more time, based on findings from the IBM System Sciences Institute.
Choosing the right CI tools is key, like Jenkins or Travis CI, which can automate tests and deployments. Automated testing isn't just about speed; it covers a range of practices like keyword-driven, data-driven, and modular testing frameworks to improve app quality and accuracy.
As Martin Fowler, a CI guru, said,
"Continuous Integration doesn't get rid of bugs, but it does make them dramatically easier to find and remove."
Using test automation tools is a strategic move that directly benefits your code's structure, maintainability, and performance, making it essential for modern development workflows.
Refactoring for Code Maintainability
(Up)Refactoring is a big deal in coding, and it's all about restructuring the existing code without messing with how it works on the outside. The main goal is to make the code easier to maintain and modify.
According to some research, refactoring can reduce code complexity by around 5.2%, which is a game-changer when it comes to keeping things manageable.
When you're working on a massive project, refactoring is a must-have, as it directly impacts how scalable and adaptable your system can be. It's also a handy way to deal with code smells, like duplicated or overly complicated methods, which are red flags that your codebase needs some TLC.
Now, when it comes to tackling big codebases, you gotta have a strategy:
- Regular Code Reviews: Get your team on board with regular code reviews, and you'll be able to spot opportunities for refactoring, prevent code rot, and encourage everyone to take ownership of the code, as industry insiders suggest.
- Automated Testing: Back up your refactoring with a solid suite of automated tests to ensure everything still works as intended, giving you the confidence to make changes without breaking stuff.
- Incremental Changes: Take it step-by-step instead of trying to refactor everything at once. Gradual changes make it easier to integrate and test, reducing the risk of major issues.
So, when should you refactor? Duplicated code, overly complex methods, or changes in requirements or technology are good indicators.
Here's a quick rundown of the process:
- Identify the code sections that need some love.
- Make sure you have a solid testing framework in place to catch any regressions.
- Break down the refactoring into manageable tasks.
- Refactor bit by bit, regularly verifying that everything still works as expected.
- Review the refactored code with your team to ensure clarity and maintainability.
As Martin Fowler, a refactoring guru, said, "Any fool can write code that a computer can understand.
Good programmers write code that humans can understand." That's the essence of refactoring – creating a codebase that's readable and extensible.
Teams that prioritize refactoring are building a solid foundation, ensuring their software can adapt to changing user needs and technological advancements without falling apart.
It's a long-term investment that pays off big time.
Scaling and Performance Considerations
(Up)Scaling up big projects is a real pain in the ass, mainly due to the code getting all tangled up and having to keep things running smoothly for all the users.
One of the biggest hurdles is making sure the user experience stays lit as more people hop on board, which often requires some performance optimization tricks.
For instance, implementing caching mechanisms can cut those load times down by like 50%, and optimizing your database queries can make things way faster and more efficient, especially when you're dealing with a metric ton of data.
Organizing all that code to handle the growth requires some serious strategy, like the ideas from Martin Sandin's strategies for keeping your code tidy.
Separating your code into different components can make sure everything stays tight and organized.
- Modular Design: Split your codebase into separate modules that can be managed and scaled up independently, so each module only has the stuff it needs and exposes the right functionalities.
- Service-Oriented Architecture (SOA): Use services to separate concerns and make things more scalable, which also makes it easier to update and maintain stuff, just like Unity's suggestions for growing projects.
- Load Balancing: Spread out the traffic across multiple servers to keep things stable when everyone's jumping on at once, and also use methods like serverless functions for even more scalability.
The best ways to keep your code quality on point while scaling up involve continuous testing and integration, which keeps your codebase solid and agile.
Stats show that implementing continuous testing can reduce post-deployment errors by up to 75%. Plus, performance profiling is key, helping you identify bottlenecks and areas that need improvement, which can lead to some serious performance gains.
The experts say, "It's not just about writing more code, it's about organizing the code for optimal performance and scalability." Making sure you've got a structured approach to scaling means doing regular code refactoring, keeping your codebase clean and efficient by getting rid of redundancies and making it easier to read.
It's not just about writing more code, it's about organizing the code for optimal performance and scalability.
Having solid code documentation is crucial, as it serves as a guide for current and future devs, making it way easier to scale and maintain things.
By following these strategies and techniques backed by data, from various thought leaders and community discussions, organizations can tackle the challenges of scaling up big projects, while optimizing performance and keeping their code organization on point.
Team Collaboration and Project Management Tools
(Up)In this wild world of coding collabs, picking the right tools can be a game-changer for your squad's productivity and code organization. Project management tools are where it's at when it comes to keeping that dev grind on track.
The 2023 scene is stacked with dope tools designed to streamline your workflows, manage those codebases, and keep the communication flowing. Like, did you know that using tools like Jira, monday.com, and Asana can boost your project's efficiency by up to 25%? That's crazy! These bad boys give you visual boards for task organization, feature prioritization, and progress tracking—essential for keeping a bird's-eye view of those massive projects.
The benefits of collab tools on software project productivity are no joke.
Platforms like Slack and Google Workspace keep the communication game strong within your dev squad, integrating directly with project management software to keep everyone in the loop with real-time updates.
Here's a rundown of some of the hottest project and code management tools that are straight-up fire for enhancing team collaboration:
- GitHub: Known for its version control skills and fostering open-source collabs. It's all about code review, pull requests, and a collaborative workflow.
- GitLab: Combines continuous integration/delivery with management features to cater to all phases of the dev lifecycle and amplify your team's collaborative efforts.
- Confluence: Used as a collab wiki tool, it helps teams share knowledge efficiently and integrates seamlessly with other dev tools like Jira.
"By harnessing the power of these comprehensive tools, teams can significantly streamline their dev processes, ensuring that every line of code falls perfectly into place," says Elizabeth Thomas, a senior dev from a top tech firm.
Data shows that teams using a combo of these tools have seen a decrease in miscommunication errors by as much as 22%, emphasizing their critical role in those large-scale software endeavors.
At the end of the day, the convergence of project management tools with code organization best practices forms a synergy that's straight-up essential for the success of complex software projects.
Conclusion: Synthesizing Best Practices for Code Organization
(Up)When you're working on a massive coding project, you gotta have your shit together, ya feel me? That's where organizing your code comes in clutch.
By studying the structure and standards used by the pros, you can keep your codebase easy to maintain and ready to scale.
Modular design is the way to go.
Keeping your code modular makes it easier to spot and fix errors, helps you learn faster, and even makes your tools work better. And don't forget about naming conventions – clear and logical naming styles are crucial for making your code readable and understandable.
Documenting your code is also a game-changer.
Sites like dbt Developer Hub have the lowdown on how to keep your team on the same page and make sure everyone knows what's going on.
Staying organized is key if you want to hit those deadlines, ya dig?
When it comes to managing your code, tools like Git are your best buds. Version control makes it a breeze to integrate code without messing everything up.
And don't sleep on automated testing and continuous integration – they'll keep things running smooth and catch errors before they become a problem. The Stack Overflow crew knows what's up when it comes to code reviews, too.
Performance optimization is also crucial for scaling your project and keeping those load times lightning-fast.
Smart data structures and techniques can make a huge difference in how your app runs and feels for users.
And let's not forget project management tools! Agile and Scrum are game-changers for keeping your team on track.
Going from solving problems solo to tackling projects as a squad is a big deal, and these tools make it happen.
At the end of the day, if you want your project to stay agile, maintainable, and rock-solid as it grows, you gotta nail down those foundational code organization practices.
That's the key to success in modern software development!
Frequently Asked Questions
(Up)Why is code organization crucial in large projects?
Code organization in large projects is crucial for operability and maintainability. Automated testing & modular design lead to fewer defects. Standardized code quality reduces operational risks. Addressing bugs during design lowers costs.
What are the key best practices for code organization in large projects?
Best practices for code organization in large projects include modularity, naming conventions, documentation, version control, automated testing, and collaboration tools.
How does modularity in code design enhance software efficiency?
Modularity in code design improves code reusability, maintainability, and scalability. It enables isolated changes within modules, minimizes interdependencies, and supports easy integration of new functionalities.
Why are naming conventions and standards important in software development?
Naming conventions and standards are vital in software development as they enhance code clarity, maintainability, and ease of understanding. Consistent and descriptive names improve code readability and facilitate teamwork.
How can automated testing and continuous integration benefit code organization?
Automated testing and continuous integration boost efficiency, reliability, and code organization. They lead to higher defect detection rates, streamlined merge-to-deployment cycles, and quicker bug identification and resolution.
You may be interested in the following topics as well:
Align your personal development goals with the right programming language for a more prosperous career.
A detailed analysis brings to light the intricate parity between PHP and Ruby, each with its unique strengths in Full Stack scenarios.
Understanding the nature of state in web applications is crucial for developers seeking to build interactive and dynamic user experiences.
Factor in team expertise to ensure smooth development and long-term maintainability of your software.
Learn the significance of a High Level of Security in web frameworks, and how Django ensures your digital assets stay protected.
The integration of AR/VR in mobile apps is shaping the future of user engagement and interaction.
Find out how TypeScript enhancing JavaScript can lead to more robust and feature-rich web applications.
Ludo Fourrage
Founder and CEO
Ludovic (Ludo) Fourrage is an education industry veteran, named in 2017 as a Learning Technology Leader by Training Magazine. Before founding Nucamp, Ludo spent 18 years at Microsoft where he led innovation in the learning space. As the Senior Director of Digital Learning at this same company, Ludo led the development of the first of its kind 'YouTube for the Enterprise'. More recently, he delivered one of the most successful Corporate MOOC programs in partnership with top business schools and consulting organizations, i.e. INSEAD, Wharton, London Business School, and Accenture, to name a few. With the belief that the right education for everyone is an achievable goal, Ludo leads the nucamp team in the quest to make quality education accessible