How to manage a database in web applications?
Last Updated: June 5th 2024
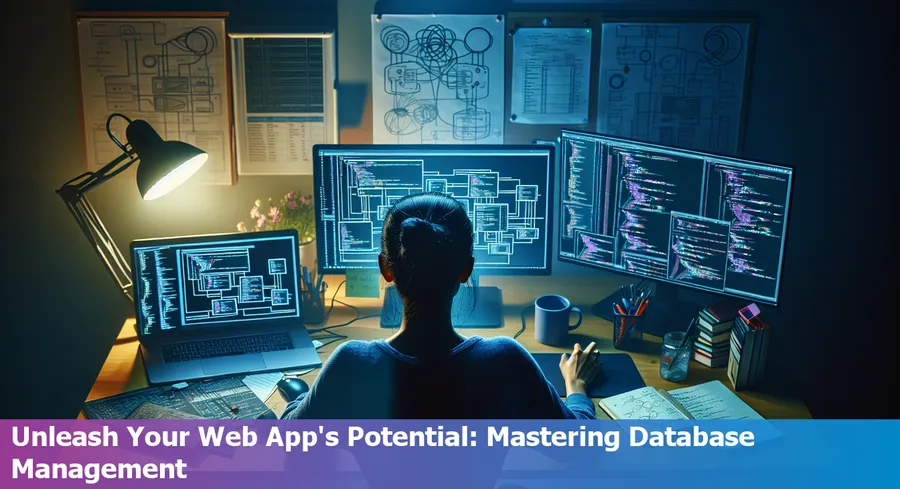
Too Long; Didn't Read:
Databases are pivotal in web apps, influencing user experience and security. Learn to choose, design, and connect databases. Optimize performance, security, scale, and monitor databases for robust web applications. Explore ORM, CRUD, queries, security practices, scaling strategies, and monitoring tools for efficient database management.
Let's talk about databases in web dev, aight? These bad boys are basically the foundation for storing and managing all the juicy data that makes websites tick.
At the core of any web app, there are three major layers: the front-end (the pretty UI you see), the back-end (the brains behind it all), and the data layer where the database lives.
When you interact with a website, say, by creating a new account or updating your profile, that's where databases come into play.
They process requests from the back-end, allowing you to create, read, update, and delete data (CRUD operations, for the nerds out there). The real MVP in this game is the Database Management System (DBMS), which ensures that your data is secure, reliable, and efficient.
Proper database management can seriously impact your user experience by speeding up data transactions, allowing the website to scale as more data comes in, and keeping your precious info safe from any potential breaches or losses.
It's not just a necessary evil; it's a strategic asset for any legit company.
With humans producing mind-boggling amounts of data every single day, knowing how to manage databases is a critical skill in web dev.
We'll dive deeper into the principles and strategies for optimizing databases in web apps, so you can build robust, secure, and high-performing services. That's what Nucamp is all about – giving you the skills to slay the full stack, including database management, so you can crush it in the dev world.
Table of Contents
- Choosing the Right Database for Your Web Application
- Designing Your Database Schema
- Connecting Your Web Application to the Database
- CRUD Operations in Web Databases
- Optimizing Database Performance
- Database Security Considerations
- Scaling Your Web Application Database
- Monitoring and Maintenance of the Database
- Conclusion and Best Practices
- Frequently Asked Questions
Check out next:
Explore how Microservices architecture is revolutionizing backend development by offering modular and scalable solutions.
Choosing the Right Database for Your Web Application
(Up)Picking the right database for your web app is like deciding what kind of wheels to put on your ride - it's a big deal. The choice depends on what your app needs when it comes to scalability, speed, and how the data is structured.
First things first, you gotta figure out if the database can handle the way you need to store and process data, and if it plays nice with the other tech you're using.
If your app needs to deal with complex transactions and keep data squeaky clean, SQL databases like PostgreSQL and MySQL are usually the go-to.
But if you need to scale up big time and handle messy, unstructured data, NoSQL databases like MongoDB and Cassandra are the MVPs.
In this day and age, making the right call on databases is crucial for performance and longevity.
The experts say you should really dig into the modern options and think about scalability; how well the database can handle more and more traffic is key.
Whether to go SQL or NoSQL might come down to how strict you need to be with data consistency and transactions.
While SQL databases like MySQL are still popular for structured data, NoSQL solutions like MongoDB are killing it when it comes to dealing with more flexible, semi-structured or unstructured data.
The word on the street is that the future might be in using both SQL and NoSQL databases strategically, like
"The future of web application development may very well lie in the strategic use of both SQL and NoSQL databases,"
so you can optimize the tech based on what you actually need, instead of trying to make one size fit all.
This "polyglot persistence" approach is all about using the right tool for the right job, kind of like how Nucamp Coding Bootcamp customizes their offerings.
At the end of the day, choosing a database isn't just important, it should align with how your app is going to grow, making sure the tech enhances the performance and user experience instead of holding it back.
Designing Your Database Schema
(Up)Getting your database set up right is key for your web app to run smoothly. You don't want your user stuck waiting for pages to load because your data's all messed up.
That's where database design comes in.
Basically, you want your data organized in a way that makes sense, and can handle whatever you throw at it. Microsoft's got some solid tips on how to keep things consistent and scalable.
Consistency helps keep your data accurate, and makes it easier to develop and maintain your app. And this thing called normalization is crucial for avoiding duplicate data and messy dependencies.
But sometimes, you might want to denormalize your data a bit, to boost read performance for apps that handle a ton of read requests.
Indexing's another trick that can seriously speed things up – like 70% faster according to some peeps on Reddit.
Just make sure your database structure matches your app's logic, use joins wisely, and plan for growth.
As that computer scientist Edgar Codd said, "Data dominates.
If you can't understand the data, you can't understand the application." A well-designed database is the foundation for a killer web app. Take the time to get it right, and your app will thank you.
Check out Vertabelo's guide for some pro tips on nailing that database design process.
Connecting Your Web Application to the Database
(Up)Connecting your web app to the database is a big deal, right? You gotta choose the right method based on what kinda data you're dealing with, how much of it there is, and how fast you need it to be.
One slick move is using connection pools, which reuse a bunch of pre-made connections for efficiency.
Or, you could go with APIs for extra security and scalability.
ORMs are the real MVPs! They automate CRUD operations, making your code less cluttered and saving you time.
Plus, they got your back by using predefined methods that protect against SQL injection attacks, which are a serious threat to web apps.
You gotta have a solid security plan too.
Use parameterized queries, encrypted connections like SSL/TLS, and lock that access down with multi-factor authentication. Studies show that apps with multi-factor auth are 96% less likely to get hacked!
While ORMs are super versatile, direct connections are the way to go for complex transactions, boosting performance by up to 25%.
According to the latest research, striking a balance between ORMs and direct connections, while following security best practices, is the key to building successful web apps.
So, stay on top of your game, and you'll be crushing it in no time!
CRUD Operations in Web Databases
(Up)Let me break it down for you about this CRUD stuff.
CRUD stands for Create, Read, Update, and Delete. It's basically how we handle data in web apps, making sure everything stays fresh and dynamic when users interact with the app.
These operations are linked to HTTP methods, like Create is POST, Read is GET, Update is PUT or PATCH, and Delete is DELETE requests. Most web apps, like 83% of them, use CRUD as their main way to deal with data.
So, you gotta master this if you want to be a dev.
Here are some best practices you should keep in mind for CRUD:
- Do atomic transactions to keep data integrity when creating or updating data.
- Use pagination when reading data to avoid performance issues with huge databases. Stackify explains why this is important for database operations.
- Encrypt sensitive data when creating or updating to keep things secure.
- Do soft deletes where data is archived instead of being permanently deleted. This is how modern apps do it, according to Sumo Logic.
When it comes to implementing CRUD operations, using ORMs (Object-Relational Mapping) can help a lot.
These bridge the gap between object-oriented programming languages and relational databases, making it easier to work with data. Codecademy says ORMs can save you a ton of coding time.
Performance is also important, and IBM says that database indexing can really boost query speed. Google also recommends using caching to speed up read operations for frequently accessed data.
Overall, you want to balance giving users a good experience with managing resources efficiently, like this guy Daniel Gonzalez says. Taking a holistic view of CRUD operations shows how crucial it is to build responsive, secure, and efficient app infrastructure.
Optimizing Database Performance
(Up)Database optimization is like the key to keeping your web apps running smooth. First off, you gotta tweak those SQL queries like a pro. Studies show that with some fine-tuning, you can boost performance by like 50-70%.
You should avoid that 'SELECT *' noise and go for precise field selections instead. Don't sleep on INNER JOIN over WHERE, and that LIMIT statement is a game-changer for previewing query results on the fly.
, watch out for SELECT DISTINCT and those pesky wildcards at the start of strings – they're performance killers.
Indexing is another crucial piece of the puzzle.
Use those indexes right, and you can slash data retrieval times by like 100x. Just make sure to choose your indexes based on how often you're running those queries and what the execution plans are looking like.
Caching is where it's at.
It's like a cheat code for reducing database load and speeding things up by keeping frequently accessed data in a faster location. You gotta identify the high-traffic data, set the cache size just right, and sync those cache expiration policies with the data refresh rates.
Implementing a Redis cache can give your response times a solid 40% boost.
When it comes to indexing, you gotta be strategic. If your queries are crossing multiple columns, multi-column indexes are the way to go.
But don't go overboard with the indexes, or else you'll end up slowing down those updates. Gotta find that sweet spot, ya dig? Don't forget to keep those index maintenance plans on point for consistent performance.
At the end of the day, optimizing your database is like investing in your ride – it'll keep things running smooth and make your users happy.
Just remember what Ben Franklin said, "An ounce of prevention is worth a pound of cure." Stay on top of these optimization strategies, and your web apps will be killing it in no time.
Database Security Considerations
(Up)You know how important your data is, right? Like, all those apps and websites you use, they store your info in databases, and there are a bunch of hackers trying to break in and steal that stuff.
It's a never-ending battle.
The National Vulnerability Database shows how many different ways there are to hack into databases, like SQL injection, which is a common way to break into data-driven apps.
And then there are other threats like unauthorized access because of weak passwords or bad session management, or even malware infections. To protect against all that, encrypting sensitive data is crucial.
It makes your info unreadable to anyone who shouldn't have access, even if they manage to break in. You can do this with standard protocols like TLS and AES.
But encryption isn't enough.
You also need to be super careful about who can access your database and how. Following best practices like the principle of least privilege, which means giving people only the minimum access they need, using multi-factor authentication, and keeping permissions tight can make it way harder for hackers to get in.
And don't forget to keep your database software up-to-date with the latest patches, because Dark Reading says a lot of vulnerabilities come from outdated software.
Even with all those precautions, regular security audits are a must.
Studies like one from Cisco show that human error causes 95% of cybersecurity incidents, and audits can help catch those mistakes. Audits also make sure you're following data protection laws like GDPR, according to Tripwire.
Regularly checking your security keeps your protocols and policies on point.
As Bruce Schneier said, "Only amateurs attack machines; professionals target people," which means training your team on security best practices is key.
To really protect your databases, you need a multi-layered approach: technical solutions like encryption, strict access control policies, and constant vigilance through audits and training.
Encryption, access control, and audits are your best defense against the ever-growing database security threats.
Scaling Your Web Application Database
(Up)Scaling a web app's database is a pain, but it's crucial if you want that sucker to keep running smoothly as it grows. The big decision is whether to go vertical (scale up) or horizontal (scale out).
Vertical scaling means beefing up the existing server with more muscle - faster CPUs, more RAM, or extra storage. Horizontal scaling is about adding more servers to share the load and data across multiple machines, which is where sharding comes into play.
Capacity planning is key - you gotta test performance with fewer boxes and project how each box will handle the load.
To deal with massive amounts of data, devs use tricks like data normalization, efficient indexing, traffic distribution, and partitioning to keep queries running smoothly.
Normalization cuts out redundant data and keeps things tidy, while indexing speeds up query response times by reducing the amount of data that needs to be scanned.
Partitioning, which is especially useful for horizontal scaling, splits data across different tables or databases, making it easier to manage and boosting performance.
Load balancing and autoscaling can also help spread the traffic evenly and automatically adjust resources based on demand.
Big players like Twitter and Facebook have shown how horizontal scaling is a lifesaver when dealing with insane amounts of user data.
As a Twitter database architect put it,
"Scaling horizontally often becomes imperative to support the sheer volume of concurrent database operations and data volume."
When deciding between vertical and horizontal scaling, you gotta consider factors like budget, growth projections, architecture choices, and how the app is built.
Understanding these dynamics is key to having a database scaling strategy that can handle the constant flood of data in high-traffic web apps.
Monitoring and Maintenance of the Database
(Up)Regular monitoring of web apps is crucial. It's like having a secret spy keeping an eye on things, making sure everything runs smoothly for the users.
We're talking about reducing downtime, catching slow queries before they become a problem, and making sure data stays secure and uncorrupted.
Now, when it comes to choosing the right tools for database maintenance, it all depends on what your app needs.
Google Cloud Monitoring is useful if you want insights into how your app and infrastructure are performing, thanks to Google's massive backend. But there are other great tools out there too, like Oracle Enterprise Manager, phpMyAdmin, and SQL Server Management Studio.
Having the right tools in your arsenal is key for keeping those databases in tip-top shape.
As for backups, you need to follow the 3-2-1 rule: three copies of your data, two different storage media, and one offsite.
It's like having a safety net in case things go south. And don't forget to test those backups regularly to make sure they actually work. Disaster recovery planning is also a must, with automation to speed up recovery time and clear documentation to guide you through the mess after a disaster.
As they say,
"The only thing worse than no backup is a backup that doesn't work."
Combining manual oversight with automated systems is the way to go for monitoring and maintaining web databases.
It's all about striking that perfect balance between hands-on management and operational efficiency, keeping your web apps running like a well-oiled machine.
Conclusion and Best Practices
(Up)Managing a web app's database ain't just about following the rules, it's about keeping up with the times. Deciding between SQL or NoSQL is a big deal, and it depends on stuff like how your data is structured and how much you need to scale.
To get that database design on point, you gotta plan out your schema and normalize that shit.
That way, you avoid performance issues and unnecessary complexity. Using Object-Relational Mappings (ORMs) and efficient CRUD operations is key for managing your data like a boss.
And don't sleep on database versioning tools like Flyway – they'll help you handle schema changes in deployed apps like a champ.
Optimizing queries with caching and indexing is where the real magic happens.
That shit can cut response times down to size. And let's not forget about database security – encryption and access management are crucial. Check out Microsoft Azure's database security solutions for some serious protection against breaches and compliance issues.
As tech keeps evolving, you might wanna consider going serverless or moving to the cloud.
That'll give you the scalability and agility to handle massive datasets, plus some extra database hardening tricks. Database admins and devs these days gotta keep learning and adapt to new trends and tools.
Nucamp's articles can help you get hip to new practices like state management and web application firewalls, so your database systems are ready for whatever the future throws at 'em.
Frequently Asked Questions
(Up)What is the importance of choosing the right database for a web application?
Choosing the right database is crucial for a web application as it impacts scalability, performance, and data structure alignment. SQL databases like PostgreSQL and MySQL are suitable for complex transactions, while NoSQL databases like MongoDB are ideal for scalability and flexible storage.
How does database schema design influence web application performance?
A well-designed database schema enhances data storage efficiency and retrieval speed. Principles like normalization reduce redundancy and dependency, while indexing can significantly improve query efficiency by up to 70%.
What are the best practices for connecting a web application to a database?
Establishing a secure and efficient connection involves choosing the right method, such as using ORMs and APIs. Security measures like parameterized queries, encrypted connections, and multi-factor authentication are essential to safeguard against SQL injection attacks and unauthorized access.
How do CRUD operations impact data management in web applications?
CRUD operations (Create, Read, Update, Delete) are fundamental for data interaction in web apps, mapping to HTTP methods like POST, GET, PUT/PATCH, and DELETE. Executing atomic transactions, employing pagination, encrypting sensitive data, and implementing soft deletes are essential best practices for effective CRUD operations.
What are the key strategies for optimizing database performance in web applications?
Optimizing database performance involves careful tuning, precise field selections, using INNER JOIN, LIMIT statements, and efficient indexing. Caching strategies, proper database indexing, and load balancing can significantly enhance query response times and overall performance.
You may be interested in the following topics as well:
Learn the art of Error Handling in APIs to enhance user experience and system reliability.
Integrating DevOps practices with microservices enhances the efficiency and speed of product deployment.
Strategize how to effectively manage high traffic volumes to ensure a smooth user experience.
Moving beyond authentication, learn the essentials of integrating authorization into your web applications for fine-grained access control.
Navigate the landscape of development by understanding the common hurdles inherent in Node.js, and how to effectively overcome them.
Implement load balancing techniques to efficiently distribute traffic and optimize resource utilization.
Discover how backend magic unfolds by understanding the contrast with client-side scripting that makes websites interactive and dynamic.
Stay ahead of the curve by understanding the latest database technology trends that are shaping the future of application development.
Ludo Fourrage
Founder and CEO
Ludovic (Ludo) Fourrage is an education industry veteran, named in 2017 as a Learning Technology Leader by Training Magazine. Before founding Nucamp, Ludo spent 18 years at Microsoft where he led innovation in the learning space. As the Senior Director of Digital Learning at this same company, Ludo led the development of the first of its kind 'YouTube for the Enterprise'. More recently, he delivered one of the most successful Corporate MOOC programs in partnership with top business schools and consulting organizations, i.e. INSEAD, Wharton, London Business School, and Accenture, to name a few. With the belief that the right education for everyone is an achievable goal, Ludo leads the nucamp team in the quest to make quality education accessible