Node.js Fundamentals: Beginner's Guide to Server-Side JavaScript
Last Updated: June 5th 2024
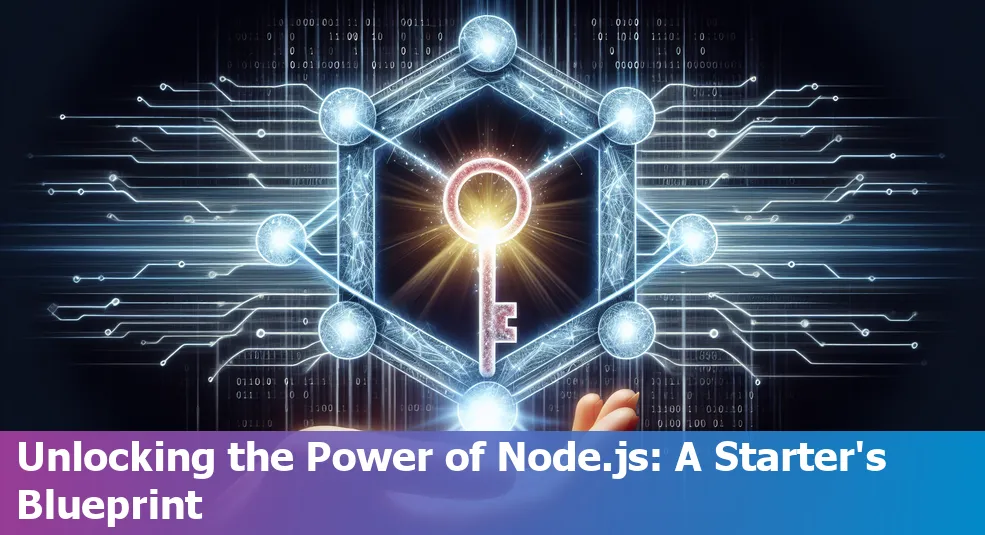
Too Long; Didn't Read:
Node.js introduced in 2009 revolutionizes server-side JavaScript with Google V8 engine for efficient event-driven architecture. Node.js's non-blocking I/O manages concurrent connections efficiently. Its popularity highlighted by top technology ranking and adoption by Netflix & PayPal. Enhance skills with Node.js Fundamentals for robust web development.
Let me tell you about this badass thing called Node.js. This baby was born in 2009 by some dude named Ryan Dahl, and it's like a game-changer for web development.
Imagine being able to run JavaScript outside of your browser, thanks to Google's V8 engine. That means you can handle multiple client requests like a boss, without getting bogged down by those old, slow server-side languages that choke on asynchronous processing.
Node.js is like the cool kid in school, always ranking high in those StackOverflow Developer Surveys for web frameworks.
And you know what else? It's besties with JSON, which is super useful for web API integration. Speaking of APIs, Nucamp's API Integration guide has got you covered if you want to dive deeper into that topic.
Node.js has this huge ecosystem called npm, which is like the biggest software registry ever, with a ton of libraries that make complex tasks a breeze.
Big shots like Netflix and PayPal have jumped on the Node.js bandwagon, experiencing insane improvements in startup times and page response times compared to their Java counterparts.
Learning Node.js isn't just about following the latest trend; it's about unleashing the full potential of server-side JavaScript, which is a crucial skill you'll learn in Nucamp's Full Stack Web + Mobile Development bootcamp.
So, what are you waiting for? Get on board and join the Node.js revolution!
Table of Contents
- How Node.js Works
- Setting up Node.js
- Creating Your First Node.js Server
- Node.js Core Modules
- Node.js Frameworks
- Exception Handling in Node.js
- Concluding Node.js for Beginners
- Frequently Asked Questions
Check out next:
Navigate the complexities of the Internet with our comprehensive Back-End Web Development Guide designed for novice coders.
How Node.js Works
(Up)Let me break it down for you in a way that's easy to understand. Node.js is all about handling multiple tasks at once without slowing down your system. It's like having a super-efficient personal assistant that can juggle a bunch of different jobs simultaneously.
At the core of Node.js, there's this thing called an event-driven architecture and non-blocking I/O model.
Basically, it means that Node.js can handle multiple operations at the same time without getting bogged down like traditional systems. It's like having a boss that can assign tasks to different employees without waiting for one person to finish their work before moving on to the next task.
Node.js works on a single-threaded event loop, which takes advantage of the system's non-blocking nature to execute operations.
It's like having a personal assistant who can handle multiple requests without getting overwhelmed. When you need something done, like loading a file or making a network request, Node.js uses callbacks to efficiently manage these events, allowing it to handle a ton of connections simultaneously without breaking a sweat.
This event-driven approach is a game-changer when it comes to performance, especially under heavy loads.
Node.js applications can handle a massive amount of traffic with low latency, making it perfect for real-time applications that need to be lightning-fast.
The non-blocking I/O model is the key to Node.js's scalability.
Here's how it works:
- While waiting for previous I/O tasks to finish, the server can process new requests,
- This means the CPU is optimally used, and no resources are wasted,
- As a result, a Node.js server can handle thousands of concurrent connections like a boss.
That's why major companies like Netflix and PayPal have jumped on the Node.js bandwagon.
PayPal reported a 35% decrease in average response time after switching to Node.js, which just goes to show how powerful this platform is.
The non-blocking I/O model's ability to handle massive amounts of data and users while keeping things running smoothly is a game-changer for modern web services.
Not only did it boost performance metrics for the big guys, but it also made life easier for developers writing server-side code.
One example of how Node.js does its event-driven magic is the EventEmitter class, which is used by various core modules.
Instead of constantly checking for updates like a paranoid freak, developers can set up event listeners and trigger callbacks when something happens. This approach avoids the inefficient polling of traditional systems and lays the foundation for responsive, data-intensive, real-time applications that can run on multiple devices simultaneously.
Setting up Node.js
(Up)Setting up Node.js is a must if you wanna get serious with server-side JavaScript. It works on pretty much any platform, and the installation process is a breeze.
If you're on Windows, check out how to install Node.js and NPM – it walks you through everything from downloading to verifying it's working properly.
For Mac and Linux users, it's a similar deal – just grab the installer from the Node.js website. The general consensus is that the default settings are good enough for most setups.
And for all you Linux geeks out there, especially the Ubuntu crew, installing Node.js is a piece of cake:
- Step one is updating your system. Refresh your package manager with
sudo apt-get update
. - Next up, installing Node.js. Get that bad boy installed by running
sudo apt-get install nodejs
. - Last but not least, verifying the installation. Make sure it's all good with
node -v
, which will show you the installed Node.js version.
Once you've got Node.js up and running, setting up your dev environment is key.
Here are some essential tips:
- The Node Package Manager (npm), the heart and soul of Node.js, usually comes bundled with the installation, but double-check with
npm -v
. - Dealing with multiple Node.js versions? Use Node Version Manager (NVM), a lifesaver for devs juggling different projects.
- It's crucial to update your PATH environment variable to include the path to your Node.js binaries.
As the experts say,
"Starting with a solid environment is key to nailing Node.js apps."
By following these basic Node.js setup steps, you'll be well on your way to server-side JavaScript mastery, avoiding common pitfalls and ensuring your server is ready to handle dynamic web apps like a boss.
It's no wonder getting this setup down is like a rite of passage into the world of Node.js, laying the foundation for exploring its vast and ever-growing ecosystem.
Creating Your First Node.js Server
(Up)Let's talk about building a basic Node.js server, 'cause that's a skill every aspiring web dev needs to have on lock. Node.js is the king of handling async I/O operations, making it a boss for developing scalable network applications.
As the Node.js intro says, it lets you write server-side code using JavaScript, leveraging the V8 engine outside of the browser.
The DigitalOcean tutorial is a real MVP, teaching beginners how to create and manage routes using the 'http' module.
Before you can start cooking with Node.js, you gotta understand the HTTP protocol – that's the backbone of data communication on the web.
HTTP requests are how browsers talk to servers, and in Node.js, you handle them by creating an HTTP server that listens for requests on a specific port.
Here's a step-by-step guide to setting up a basic Node.js server:
- Install Node.js and make sure it's all good by checking the Node and NPM versions.
- Import the 'http' module and use
http.createServer()
to handle HTTP requests and responses. - Create server logic within the 'createServer' method to manage different requests, like serving HTML content using the 'fs' module for file-based responses.
- Start processing incoming requests by having the server listen on a specified port, handling GET, POST, and other HTTP methods.
For example, managing a GET request might involve checking the request URL and responding with appropriate content, as shown in an example from Tutorials Teacher.
This transparent approach to creating a web server lets new devs easily understand HTTP methods and sets the stage for more complex applications.
Mastering HTTP requests and responses is the foundation for building more sophisticated and functional servers, allowing you to handle real-world web traffic and unlocking endless possibilities with Node.js.
Node.js Core Modules
(Up)The real power of Node.js lies in its core modules, which are like the building blocks for creating dope apps without reinventing the wheel. Modules like FS (File System), Path, and HTTP are the MVPs you need for the basic operations in Node.js.
For instance, the FS module is the key to reading and writing files, which is crucial for all those back-end processes you'll be dealing with. It's like having a boss file manager that follows the standard POSIX rules.
And the kicker, you can rock it both ways – synchronous and asynchronous. The asynchronous methods are where it's at, though, like the fs.readFile() method for reading files without blocking the program flow.
Then you got the Path module, which is your go-to wingman for handling file and directory paths.
It's like having a GPS for your code, making sure you navigate those cross-platform paths like a pro. Whether you need to normalize paths with path.normalize() or get the full address with path.resolve(), this module's got your back.
And let's not forget the HTTP module, the real MVP when it comes to networking. It's the backbone for transferring data over the web using the HyperText Transfer Protocol (HTTP).
According to the latest developer surveys, the HTTP module is a fan favorite for creating web servers, while modules like FS are killing it with file processing capabilities, letting you do everything from copying files to managing file metadata.
Using these core modules is like having a cheat code for writing clean, high-performance code.
For example, let's say you need to read some data from a file asynchronously. You'd be like const data = await fsPromises.readFile(path);
and boom, you're good to go.
Of course, you gotta keep an eye out for errors, but that's just part of the game. Bottom line, using Node.js modules is the key to unlocking the secrets of Back-End Web Development, making Node.js a must-have in your modern web app arsenal.
Node.js Frameworks
(Up)Node.js has totally revamped the server-side game, thanks to its dope frameworks that cater to all kinds of web and mobile app needs. Express.js is the OG, keeping it simple yet sturdy, and it's super popular for web and mobile apps 'cause of its minimalistic but powerful vibes Simform.
While it's a classic, devs are now exploring beyond Express, getting their hands on fresh frameworks like Koa.js and Nest.js, built for the next-gen and enterprise-level apps Inventorsoft.
Express.js is a must-have for building APIs, thanks to its efficient routing, and for Single Page Apps (SPAs) 'cause it seamlessly integrates with frontend frameworks.
In fact, the 2020 Node.js User Survey Report found that 75% of respondents were using it for its ease and robust performance. Koa.js is bringing a whole new level of expression and robustness to web apps and APIs.
By using async functions instead of callbacks, it levels up error handling and offers a fresh take on Node.js app architecture. Its strengths lie in fine-grained error handling, a modular system, and improved performance under heavy loads, making it a top pick for devs who want a contemporary, modular approach ExternLabs.
Other frameworks like Nest.js are also gaining traction due to their scalability and the ability to integrate different programming paradigms, crucial for complex systems and microservices.
The Node.js framework ecosystem is diverse, each with its own unique perks, whether it's Meteor.js for real-time apps or Socket.io for bidirectional communication essentials.
For newbies eager to dive into Node.js, there are tons of tutorials and docs across these frameworks to help you smoothly get started. As Ryan Dahl, the creator of Node.js, said, its adaptability offers a modern, comprehensible way to build servers that keep up with the ever-changing dev landscape.
Exception Handling in Node.js
(Up)Check this out! Handling errors in Node.js is crucial for keeping your app running smoothly and avoiding crashes. According to Stackify, you gotta be prepared for errors at every level of your code, and use try-catch blocks wherever there's a chance of an exception being thrown.
Runtime issues and bugs are pretty common in Node.js, so you gotta stay on top of managing them.
LogRocket's blog has some solid tips too, like separating programmer errors from operational errors, using the right error handling techniques, and dealing with errors at the appropriate level to prevent your program from crashing.
Since Node.js is non-blocking and runs asynchronous code, you'll need to use error-first callbacks, promises with .catch(), and try-catch blocks with async/await to handle errors smoothly.
StackExchange discussions highlight the importance of handling errors at the appropriate layer, so you don't spam your logs with duplicate info.
They suggest letting errors bubble up to layers that can handle them properly. Frameworks like Koa.js or Express.js have built-in error handling features that can help with this.
Unhandled exceptions can straight up terminate your Node.js process, so using process.on('uncaughtException', handler)
can act as a safety net.
But don't rely on it too much – Burke Holland recommends using middleware in Express.js to manage operational errors effectively. As the experts say, proper error management goes beyond just catching exceptions – you gotta understand how Node.js works and incorporate solid practices.
If you wanna build bulletproof apps, mastering this stuff is essential. Resources like the Nucamp Coding Bootcamp's Back-End Web Development Guide can help you dive deep into this topic.
Concluding Node.js for Beginners
(Up)Check this out! Getting into Node.js is a smart move if you want to level up your JavaScript game on the server side.
Now that we've covered the basics, it's time to plot your next steps to become a Node.js ninja. Start by nailing down the fundamentals like async programming, working with RESTful APIs, and getting a grip on the NPM ecosystem – this is key for building apps that can scale like a boss.
Once you've got that down, dive into Node.js's non-blocking operations and learn about the V8 JavaScript engine to unlock advanced skills like streaming data, microservices architecture, and managing databases (both SQL and NoSQL).
Here's a roadmap for your next steps:
- Level up your Express.js skills and master middleware.
- Get down with database integration, whether it's MongoDB or PostgreSQL.
- Explore authentication methods like JWT and OAuth2.
- Implement real-time communication with WebSocket or Socket.io.
- Dive into test-driven development (TDD) for Node.js apps.
To prove your skills and stand out, consider getting certified as a Node.js Certified Developer (NCD) by the OpenJS Foundation – it'll seriously boost your cred.
"The rise of Node.js in enterprise environments underscores the value of certification for developers," as the experts say.
Keep learning and stay ahead of the curve by checking out resources like Nucamp's Future of Web Development to stay up-to-date with trends like serverless architecture, containerization with Docker, and orchestration with Kubernetes.
This way, you'll be able to build cutting-edge, high-performance, and scalable apps that'll crush the competition in this ever-evolving tech landscape.
Frequently Asked Questions
(Up)What is Node.js?
Node.js is a server-side JavaScript runtime environment introduced in 2009 by Ryan Dahl. It utilizes Google's V8 engine for efficient event-driven architecture and non-blocking I/O to handle multiple operations simultaneously.
How does Node.js handle multiple concurrent connections efficiently?
Node.js operates on a single-threaded model using non-blocking I/O, allowing it to process new requests while waiting for previous I/O tasks to finish. This optimally uses CPU time, enabling Node.js to handle thousands of concurrent connections efficiently.
What are some popular Node.js core modules?
Some popular Node.js core modules include 'fs' for file system operations, 'path' for working with paths, and 'http' for networking support over HTTP. These modules provide essential functionalities for building efficient applications.
Which Node.js frameworks are commonly used for server-side development?
Express.js is a popular Node.js framework known for its simplicity and robustness, ideal for web and mobile applications. Other frameworks like Koa.js and Nest.js offer tailored solutions for different development needs.
Why is effective exception handling important in Node.js?
Effective exception handling in Node.js is crucial for ensuring application stability and preventing server crashes. Proper error management techniques like try-catch, promises, and asynchronous functions help streamline error handling in asynchronous code execution.
You may be interested in the following topics as well:
Take the first step towards expertise with our PHP Scripting Walkthrough, designed for the aspiring coder.
Understanding the intricate dance of user's requests and server's responses is vital for any developer looking to build responsive and efficient web services.
Find easy-to-follow steps on how to install MySQL and prepare your development environment for success.
Embark on the journey of mastering web development frameworks to unlock new opportunities in the tech industry.
Begin your journey in building your first Progressive Web App with our step-by-step guide.
Become captivated by the intricacies and Journey into backend development, where code meets functionality in the most fascinating ways.
Chevas Balloun
Director of Marketing & Brand
Chevas has spent over 15 years inventing brands, designing interfaces, and driving engagement for companies like Microsoft. He is a practiced writer, a productivity app inventor, board game designer, and has a builder-mentality drives entrepreneurship.